Opencart API documentation for the developer: supports output formats that return a JSON response. Output formats are defined by specifying the output request parameter. With opencart API we can perform CRUD functionalities.
Opencart 4 API documentation
In Opencart 4 there are some changes on the API endpoints, here is the login API endpoint /index.php?route=api/account/login:
Here is the POSTMAN testing:
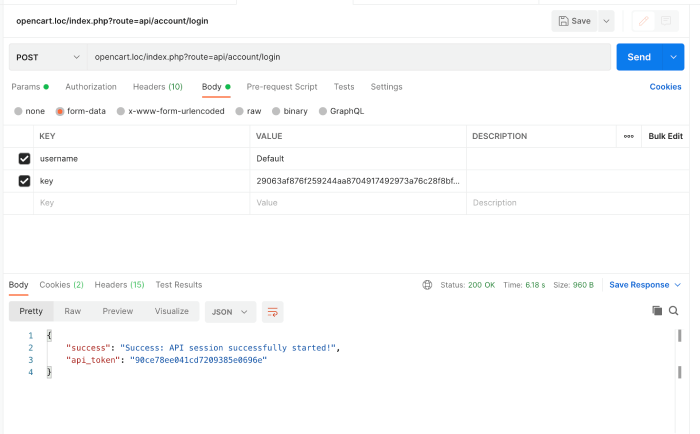
Here are the API username and API key in the Opencart admin.
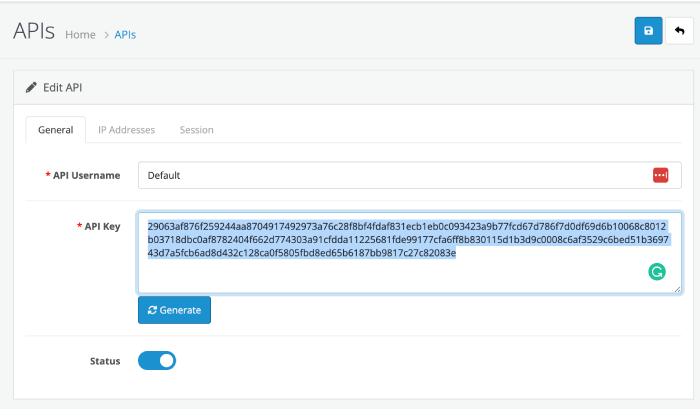
IP Addresses to allow to access the Opencart API endpoints:
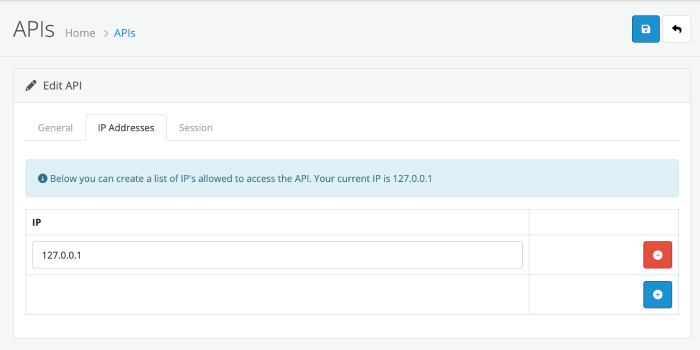
If you are not using the master branch and using the Opencart 4.0.1.1 then your endpoints may not work, and looking at the code there is a session of api_id that is checked and that session is not set. So there are some issues, so to make it work, we uncomment the following lines of code at catalog/controller/startup/api.php
<?php
namespace Opencart\Catalog\Controller\Startup;
class Api extends \Opencart\System\Engine\Controller {
public function index(): object|null {
if (isset($this->request->get['route'])) {
$route = (string)$this->request->get['route'];
} else {
$route = '';
}
// if (substr($route, 0, 4) == 'api/' && $route !== 'api/account/login' && !isset($this->session->data['api_id'])) {
// return new \Opencart\System\Engine\Action('error/permission');
// }
return null;
}
}
Example of Opencart API call
Here is an example of an Opencart API call
<?php
$url = 'https://www.yourdomain.com/index.php?route=api/account/login&language=en-gb&store_id=0';
$request_data = [
'username' => 'Default',
'key' => 'YOURSECRETKEY'
];
$curl = curl_init();
curl_setopt($curl, CURLOPT_URL, $url);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($curl, CURLOPT_HEADER, false);
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, 0);
curl_setopt($curl, CURLOPT_CONNECTTIMEOUT, 30);
curl_setopt($curl, CURLOPT_TIMEOUT, 30);
curl_setopt($curl, CURLOPT_POST, 1);
curl_setopt($curl, CURLOPT_POSTFIELDS, $request_data);
$response = curl_exec($curl);
$status = curl_getinfo($curl, CURLINFO_HTTP_CODE);
curl_close($curl);
if ($status == 200) {
$api_token = json_decode($response, true);
if (isset($api_token['api_token'])) {
// You can now store the session cookie as a var in the your current session or some of persistent storage
$session_id = $api_token['api_token'];
}
}
$url = 'http://www.yourdomain.com/opencart-master/upload/index.php?route=api/sale/order.load&language=en-gb&store_id=0&order_id=1';
$curl = curl_init();
curl_setopt($curl, CURLOPT_URL, $url);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($curl, CURLOPT_HEADER, false);
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, 0);
curl_setopt($curl, CURLOPT_CONNECTTIMEOUT, 30);
curl_setopt($curl, CURLOPT_TIMEOUT, 30);
curl_setopt($curl, CURLOPT_POST, 1);
curl_setopt($curl, CURLOPT_POSTFIELDS, $request_data);
// Add the session cookie so we don't have to login again.
curl_setopt($curl, CURLOPT_COOKIE, 'OCSESSID=' . $session_id);
$response = curl_exec($curl);
curl_close($curl);
Opencart 3 API documentation
When we see API at Admin >> System >> Users >> API, then we are interested to explore it. We checked the catalog folder and found the image below:
All these tests are made in demo.webocreation.com. Checking the code we have to log in first. So we type the following URL but it gives me the following error.
https://demo.webocreation.com/index.php?route=api/login
<b>Notice</b>: Undefined index: key in <b>D:\xampp\htdocs\opencart2302\catalog\controller\api\login.php</b> on line <b>11</b>[]
Again we checked the code and found the following:
// Login with API Key $api_info = $this->model_account_api->getApiByKey($this->request->post['key']); if ($api_info) {
Which means we have to log in through the POST request. So we wrote the following curl code to log in through the POST URL.
We write the following code in opencartapi.php and upload it to webocreation.com root server:
<?php $curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => "https://webocreation.com/index.php?route=api%2Flogin", CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => "", CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 30, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => "POST", CURLOPT_POSTFIELDS => "------WebKitFormBoundary7MA4YWxkTrZu0gW\r\nContent-Disposition: form-data; name=\"key\"\r\n\r\nG63RZVjNnkPS3KdI8DZRrDph4LueZCRJcPwTfgnH8KzMFvVrsjp4g73YM1W3RdkTHRHyXK7c6vDVUsadxtx8c8r1uW2NWt0flPFNm5pVCq862jrBbrmztbBlPi4GWf9kQeB3YqT3uyOp7KldgRrvRu3eROSyGZZH2HEJ9sh9zSbXpm0u6wIOOdBoaNARzUOD74fHSn5iAYwCwPHeVkA29p3tkIPr8OFIzA9r3UGOXJ9xhWKojsGuKwnWEyaKuMB0\r\n------WebKitFormBoundary7MA4YWxkTrZu0gW--", CURLOPT_HTTPHEADER => array( "cache-control: no-cache", "content-type: multipart/form-data; boundary=----WebKitFormBoundary7MA4YWxkTrZu0gW", "postman-token: dc6b20c6-b84d-3b12-3b89-e586d0058347" ), )); $response = curl_exec($curl); $err = curl_error($curl); curl_close($curl); if ($err) { echo "cURL Error #:" . $err; } else { echo $response; }
CURLOPT_URL: OpenCart API URL
Below is the part of the code where you add your key that you have to change to yours:
name=\”key\”\r\n\r\nG63RZVjNnkPS3KdI8DZRrDph4LueZCRJcPwTfgnH8KzMFvVrsjp4g73YM1W3RdkTHRHyXK7c6vDVUsadxtx8c8r1uW2NWt0flPFNm5pVCq862jrBbrmztbBlPi4GWf9kQeB3YqT3uyOp7KldgRrvRu3eROSyGZZH2HEJ9sh9zSbXpm0u6wIOOdBoaNARzUOD74fHSn5iAYwCwPHeVkA29p3tkIPr8OFIzA9r3UGOXJ9xhWKojsGuKwnWEyaKuMB0\r\n
Then I run http://telgap.com/opencartapi.php
{"error":{"ip":"Warning: Your IP **.**.**.** is not allowed to access this API!","key":"Warning: Incorrect API Key!"}}
I add my telgap.com IP at Admin >> Users >> API >> edit >> IP Addresses tab:
After I add my IP and run the URL http://telgap.com/opencartapi.php again I got the successful message:
{"success":"Success: API session successfully started!","token":"kyJoxIKh9wEShzUxvBz2urUZSq"}
Following is the POSTMAN workout to achieve it.
I hope it will help somewhat to dig more about the OpenCart API.
You can check the following Opencart API-related posts:
- https://webocreation.com/pull-products-json-through-ap-opencart
- https://webocreation.com/opencart-api-documentation-to-create-read-query-update-and-upsert
- https://webocreation.com/opencart-api-documentation-developer
Please let us know where and how are you using the Opencart API that you have used in eCommerce websites or have any questions or suggestions, please subscribe to our YouTube Channel and read more about Opencart 4 tutorial, opencart 3 tutorials, custom module theme development. You can also find us on Twitter and Facebook.
Hey rupak , I have a query , What if a prson has a dynamic IP or What if the IP is keep on changing on every post request. How are we going to handle that.
Regards
Hi @Sushil,
May not the good Idea but you can make following changes to allow all IPs:
Go to catalog/controller/startup/session.php and comment out if ($api_query->num_rows) { statement like below:
//if ($api_query->num_rows) {
$this->session->start($this->request->get[‘api_token’]);
// keep the session alive
$this->db->query(“UPDATE `” . DB_PREFIX . “api_session` SET `date_modified` = NOW() WHERE `api_session_id` = ‘” . (int)$api_query->row[‘api_session_id’] . “‘”);
//}
With this, it will not check the listed IPs.
Hope it is helpful
Hi Rupak,
Thanks for the videos.
I am using opencart 3.0.3.2. Will that work?
how to login customer username and password ? But your article indicates it returns api token , since the login function requires 2 parameter such as username and password ? Can you please enlighten me thank you
Hi thanks for the blogs you do, they extremely helpful. When using GET to fetch orders from Opencart ( index.php?route=api/order/info ) it doesn’t return but when I add order ID it does? (index.php?route=api/order/info/order_id=81) How am I able to retrieve all the orders in a list? Your advice would be greatly appreciated. Thanks
Hi Chase, we need to create custom API to pull orders list. Right now, default Opencart API does not have an endpoint to get the orders list.
Please how did you figure this out
Hi thanks for the blogs you do, they extremely helpful. When using GET to fetch orders from Opencart ( index.php?route=api/order/info ) it doesn’t return but when I add order ID it does? (index.php?route=api/order/info/order_id=81) How am I able to retrieve all the orders in a list? Your advice would be greatly appreciated. Thanks
I Hello, I tried the way you have shown here but still I get undefined key
I Hello, I tried the way you have shown here but still I get undefined key
Hello, I have followed your steps, but this error appeared “CORS Error: The request has been blocked because of the CORS policy”. would you please advise what is the problem?
Hello,
I need your help, I am a beginner in Opencart and now I have a problem to solve, to select the product data in JSON format from Opencart 1.5
But the products must be in 2 languages, and the category must be indicated
I want to send data to this service
The data should be in this format
Thank you very much, I hope you can help me with the given problem
Hello domain.com/index.php?route=api/account/login everything is normal but I think the seo url is throwing 404. What should I do? Everything is fine in api access, but I can’t get data. The page you are looking for is not found.
If you can access lik e https://demo.webocreation.com/index.php?route=api/account/login and get {“error”:”Warning: Incorrect API Key!”} then it is accessible, if not then you may need to check the redirect code or .htaccess redirect code or similar.
.htaccess code sir
index.php?route=api/account/login
RewriteRule ^login/?$ index.php?route=api/account/login [L] #login page
this didn’t work though